How to use 3ID Connect in browser applications
Learn how to add 3ID Connect to your browser-based Ceramic app.
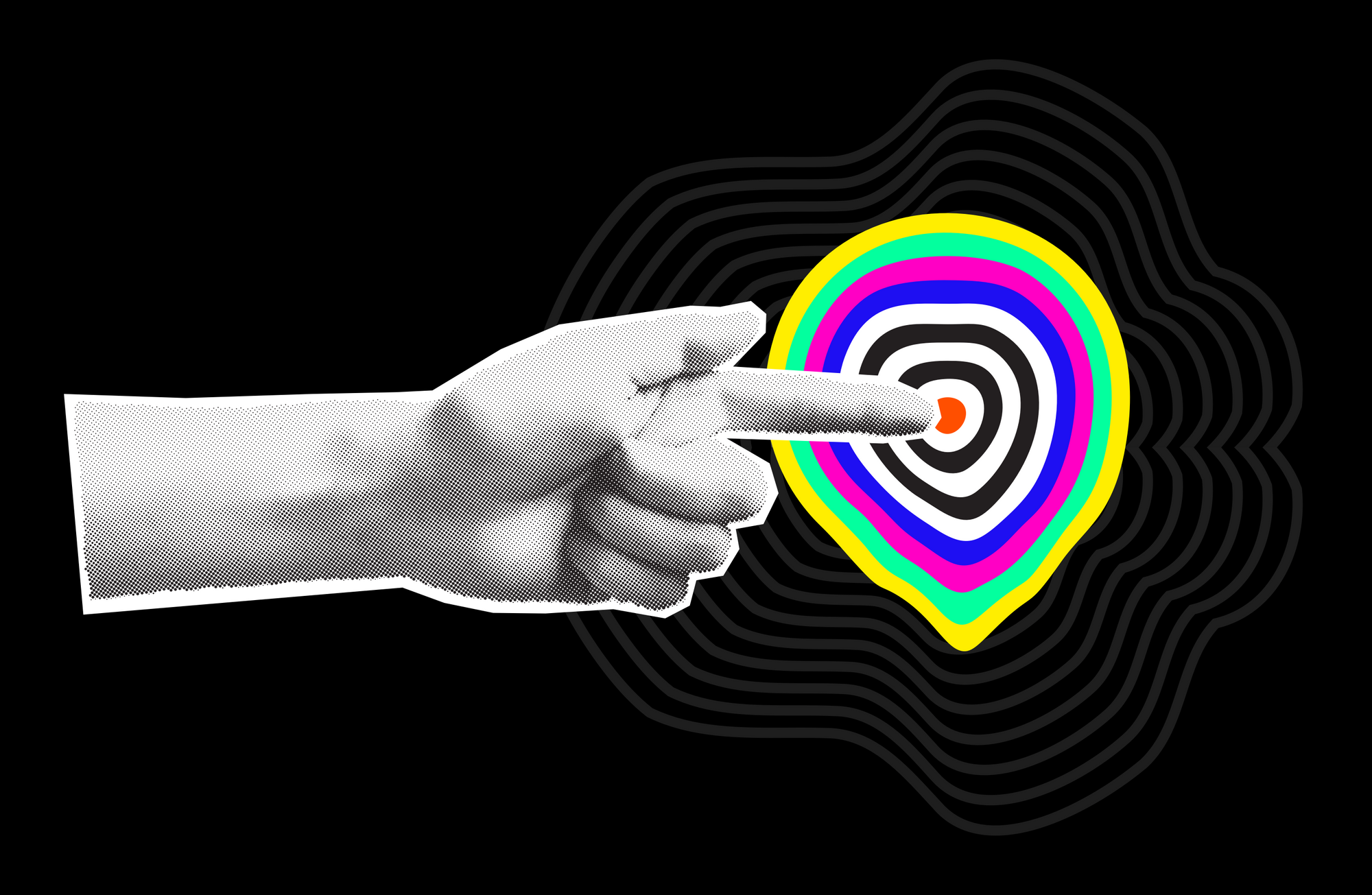
3ID Connect is a hosted 3ID identity management and authentication system for use within browser applications that allows users to use their existing blockchain accounts to onboard to applications, control/authenticate their DID, and interact with Ceramic streams. We covered a full overview of 3ID Connect, how it works, and various user flows in a previous post. You can try a simple demo of 3ID Connect in a browser application here.
This guide will walk you through how to add 3ID Connect to your browser-based Ceramic application. It is the second part of a three-part series on 3ID Connect. Other posts include:
Installation
Install 3ID Connect into your browser application using npm.
npm install @3id/connect
Usage & Authentication
During application runtime, 3ID Connect can help you onboard decentralized identities to your application while allowing your users to leverage their existing blockchain wallets to provide authentication signatures.
First, import 3ID Connect:
import { ThreeIdConnect, EthereumAuthProvider } from '@3id/connect'
Then use an AuthProvider to connect a blockchain wallet to 3ID Connect. AuthProviders typically consume a signing interface and an account or address.
const threeIdConnect = new ThreeIdConnect()
const addresses = await window.ethereum.enable()
const authProvider = new EthereumAuthProvider(window.ethereum, addresses[0])
await threeIdConnect.connect(authProvider)
In the future there will be support for AuthProviders using accounts from many different blockchains and services, however right now Ethereum is primarily supported. In the "Add authentication with new blockchains in 3ID Connect" post we detail how to add support for new blockchain accounts as AuthProviders. This would allow your users to use different blockchain wallets to authenticate 3IDs in 3ID Connect.
Application Interactions
Once connected using an authProvider
, you can begin to interact with 3ID Connect. However most often your application will not directly interact with 3ID Connect; instead you will just get a DID Provider from 3ID Connect which will allow you and your users to interact with a number of other libraries that require DID authentication, signing, encryption, etc such as Ceramic
. The library consuming the DID Provider will request authentication and permission from 3ID Connect when necessary.
const didProvider = await threeIdConnect.getDidProvider()
For example you can now instantiate js-idx
and js-ceramic
with the DID Provider and a user can create and update Ceramic streams with their DID using 3ID Connect.
import Ceramic from '@ceramicnetwork/ceramic-http-client'
import { IDX } from '@ceramicstudio/idx'
import { DID } from 'dids';
const did = new DID({ provider: didProvider, resolver: <DID Resolver> })
const ceramic = new Ceramic(<'LOCAL_CLI_NODE_OR_REMOTE'>)
await ceramic.setDID(did)
const idx = new IDX({ ceramic })
Experimenting with Ceramic
The simplest way to to get started using 3ID Connect as a DID Provider for a Ceramic node is by running a local node using the Ceramic CLI. To do this you'll need to install the Ceramic CLI and then you can create and update Ceramic TileDocuments for your application's data.
$ npm install -g @ceramicnetwork/cli
$ ceramic daemon
Once the daemon is running, the Ceramic API will be available at http://localhost:7007
.
Additional ways to install 3ID Connect
This guide demonstrates how to directly install 3ID Connect into your Ceramic application. However, 3ID Connect is also included out of the box as a dependency in the IDX Web SDK. If you're building browser-based applications and want to use the full IDX protocol for decentralized identity and user-centric data, we recommend checking it out.
Further Reading
Check out the other posts in this three-part series on 3ID Connect:
Questions?
Reach out in the Ceramic Discord.