How to migrate from 3Box to IDX for profile queries
A guide and code snippets for migrating your application from 3Box to IDX for profile queries.
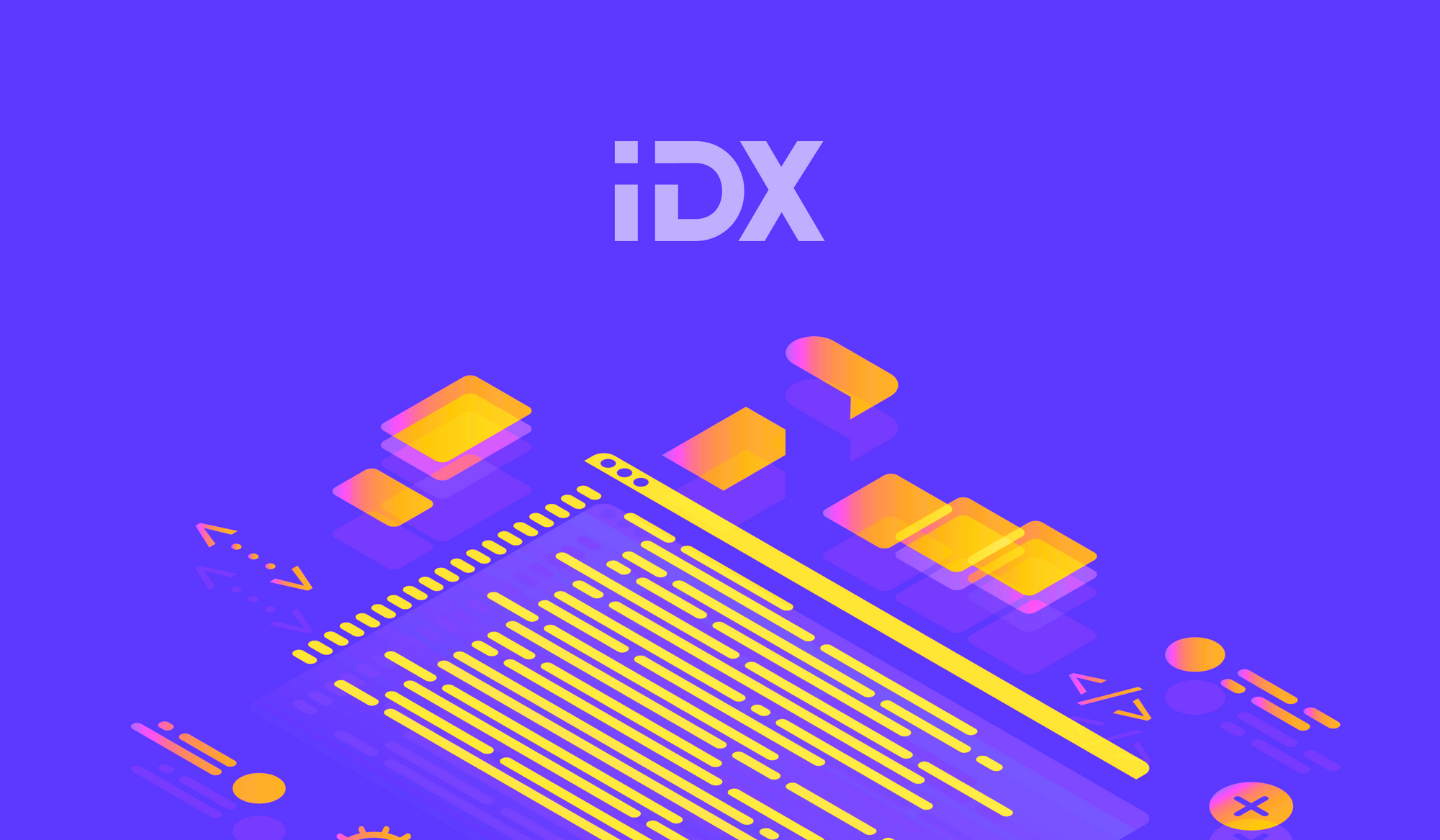
3Box Labs is sunsetting 3Box products in favor of the new and more powerful combination of Ceramic Network and the Identity Index (IDX) protocol. This post provides a short tutorial on how to update your application to load user profiles using the Self.ID SDK instead of the 3Box profiles API. Making this upgrade ensures your application will always be loading the most current profiles for your users.
Why use Self.ID instead of 3Box?
Migrating your application from using 3Box to Self.ID for fetching user profiles will ensure you are always querying up-to-date profiles for your users, whether a given user has migrated from 3Box to Ceramic/IDX or not.
As Ceramic mainnet goes live, existing 3Box users will have their profiles migrated over to IDX. Updating a user's IDX requires the user to be authenticated and sign the updates, so this will not happen all at once. Instead, the first time a user interacts with a Ceramic-enabled app that uses 3ID Connect for authentication they will be prompted to migrate their account from 3Box to IDX and the data will be transferred in the background for them.
To make sure you are accurately pulling profiles for all users, we recommend upgrading to IDX ASAP. The 3Box API will keep working for the immediate future. However, if you do not upgrade you will only load outdated profiles from the 3Box API for users who have migrated. The Self.ID API solves this by returning an IDX profile for migrated users and falling back to 3Box profiles for users who have not yet migrated.
How to query profiles with Self.ID
This tutorial will show you how to fetch profiles in order to display them in your application's UI. The code below allows your app to display an IDX profile if one exists, and if not, will fall back to displaying a legacy 3Box profile.
First, we need to install the following packages of the Self.ID SDK:
npm install @self.id/core @self.id/3box-legacy
Now let's import our dependencies:
import { Core } from '@self.id/core'
import { getLegacy3BoxProfileAsBasicProfile } from '@self.id/3box-legacy'
Now let's load a profile for a user. Notice below that we first try to load the profile using the core.get
method. This attempts to retrieve the user's basicProfile
from IDX, however if one is not present because the user has not yet migrated to Ceramic/IDX, then we can fallback to use the getLegacy3BoxProfileAsBasicProfile
function to get the user's profile from the 3Box API.
const core = new Core({ ceramic: 'https://gateway.ceramic.network' })
const ethAddress = '0xabc123...'
let profile = await core.get('basicProfile', ethAddress + '@eip155:1')
if (!profile) {
profile = await getLegacy3BoxProfileAsBasicProfile(ethAddress)
}
console.log(profile)
You may have noticed that the Ethereum address is concatenated with @eip155:1
. This is because the core.get
method can be used to lookup data for accounts on any blockchain. eip155:1
is simply the blockchain namespace for Ethereum mainnet. See the CAIP-10 spec for more details.
Questions or support?
We're always available to answer any questions and assist you through this transition period. Reach out in the Ceramic Discord for help.
Website | Twitter | Discord | GitHub | Documentation | Blog | IDX